71.Simplify Path
题目:
Given an absolute path for a file (Unix-style), simplify it.
For example,
path = "/home/"
, => "/home"
path = "/a/./../../c/"
, => "/c"
另外题目需要注意的是当出现"/../"
时,返回"/"
,
多个"/"
看成一个就行。
理解:
输入一个路径,将路径简单化,也就是去掉其中的".."
,"."
以及多余的"/"
,另外路径的最后不要加上"/"
。
代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| class Solution { public String simplifyPath(String path) { String[] strs = path.split("/"); String[] res = new String[strs.length]; int idx = 0; for ( String str : strs ) { if ( str.length() == 0 || str.equals(".") ) { continue; } else if ( str.equals("..") ) { if ( idx != 0 ) { idx--; } } else { res[idx++] = str; } } if ( idx == 0 ) return "/"; StringBuilder res_str = new StringBuilder(); for ( int i = 0; i < idx; i++ ) { res_str.append("/").append(res[i]); } return res_str.toString(); } }
|
复杂度:O(n),运行时间7ms。
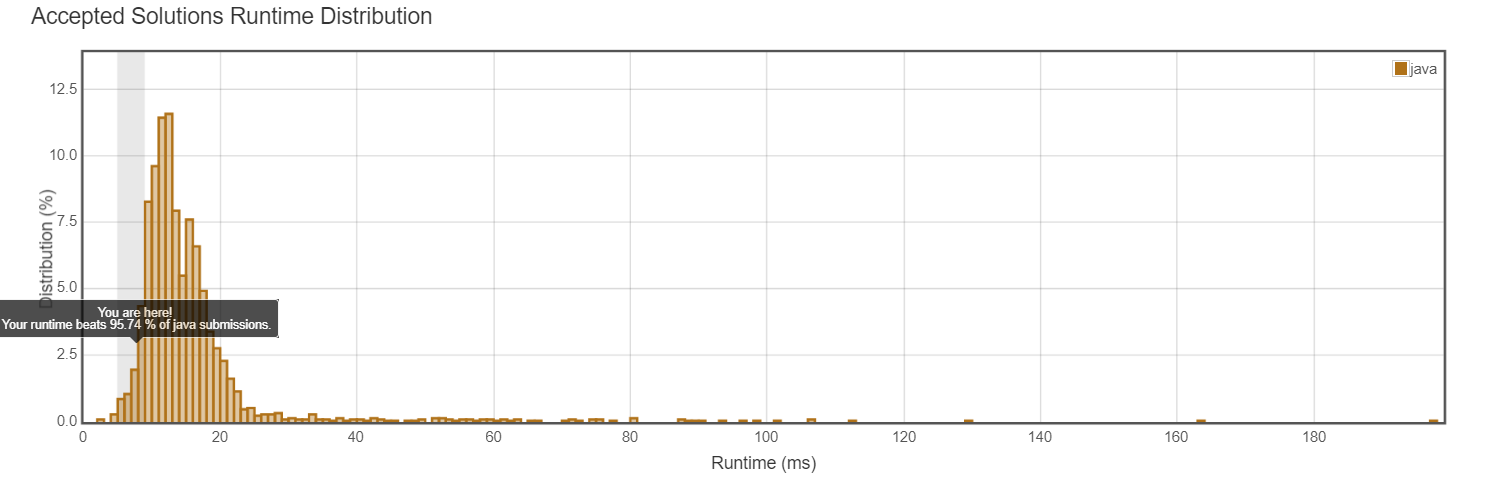
这里有一个值得注意的地方,最开始,我是这样写的:
1
| String[] strs = path.split("/+");
|
之后改成了,
1
| String[] strs = path.split("/");
|
瞬间从15ms击败25%,变成了击败95%。所以split
的多字符匹配还是要慢得多。